java设计模式示例.docx
《java设计模式示例.docx》由会员分享,可在线阅读,更多相关《java设计模式示例.docx(56页珍藏版)》请在冰豆网上搜索。
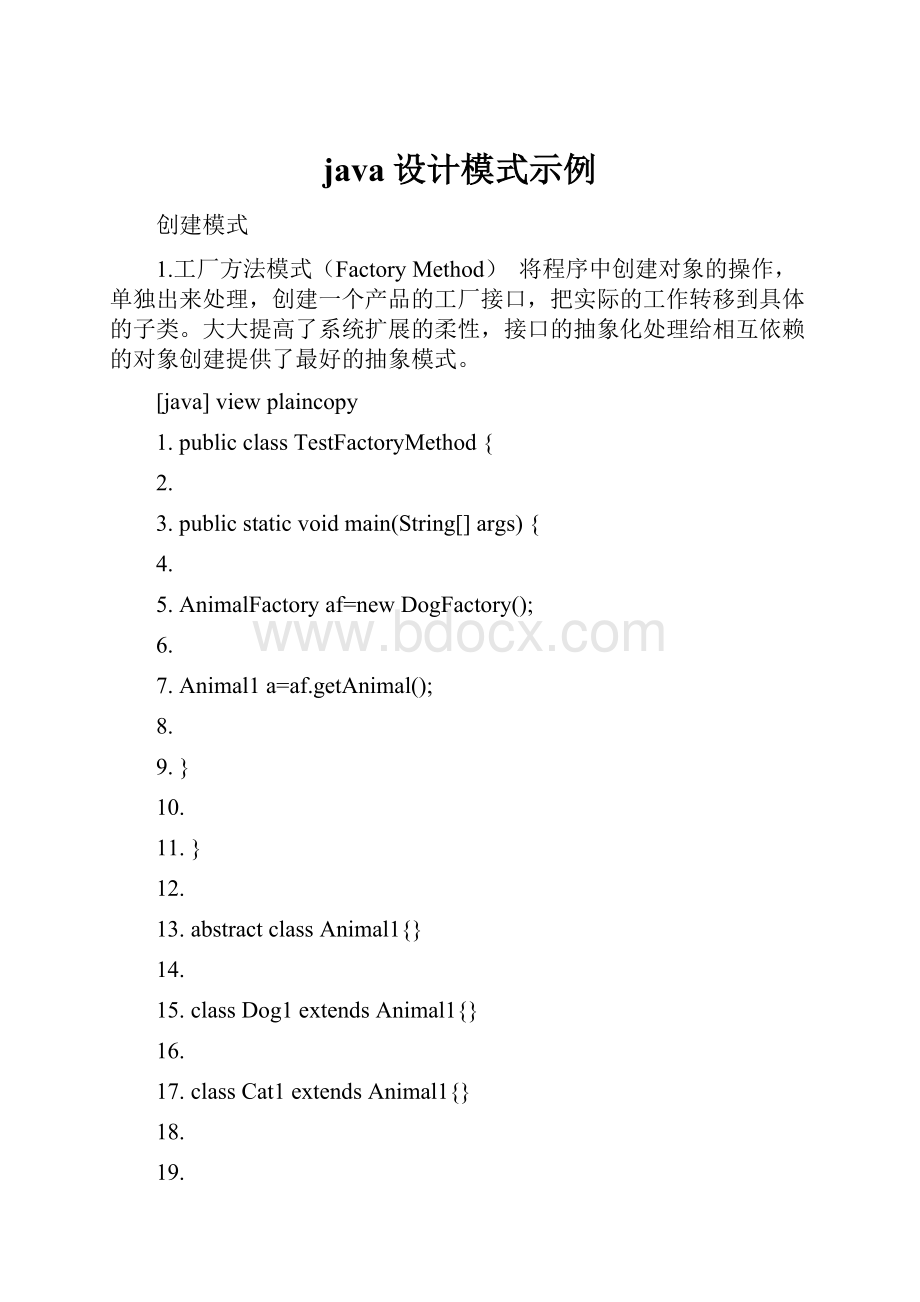
java设计模式示例
创建模式
1.工厂方法模式(FactoryMethod) 将程序中创建对象的操作,单独出来处理,创建一个产品的工厂接口,把实际的工作转移到具体的子类。
大大提高了系统扩展的柔性,接口的抽象化处理给相互依赖的对象创建提供了最好的抽象模式。
[java] viewplaincopy
1.public class TestFactoryMethod {
2.
3.public static void main(String[] args) {
4.
5.AnimalFactory af=new DogFactory();
6.
7.Animal1 a=af.getAnimal();
8.
9.}
10.
11.}
12.
13.abstract class Animal1{}
14.
15.class Dog1 extends Animal1{}
16.
17.class Cat1 extends Animal1{}
18.
19.
20.
21.abstract class AnimalFactory{
22.
23.public abstract Animal1 getAnimal();
24.
25.}
26.
27.class DogFactory extends AnimalFactory{
28.
29.public Animal1 getAnimal(){
30.
31.System.out.println("Dog");
32.
33.return new Dog1();
34.
35.}
36.
37.}
38.
39.class CatFactory extends AnimalFactory{
40.
41.public Animal1 getAnimal(){
42.
43.System.out.println("Cat");
44.
45.return new Cat1();
46.
47.}
48.
49.}
2.抽象工厂模式(AbstractFactory)针对多个产品等级的情况,而工厂方法模式针对单一产品等级的情况。
[java] viewplaincopy
1.import java.awt.*;
2.
3.import javax.swing.*;
4.
5.import java.awt.event.*;
6.
7.public class TestAbstractFactory {
8.
9.public static void main(String[] args) {
10.
11.GUIFactory fact=new SwingFactory();
12.
13.Frame f=fact.getFrame();
14.
15.Component c1=fact.getButton();
16.
17.Component c2=fact.getTextField();
18.
19.
20.
21.f.setSize(500,300);
22.
23.f.setLayout(new FlowLayout());
24.
25.f.add(c1);
26.
27.f.add(c2);
28.
29.f.setVisible(true);
30.
31.
32.
33.f.addWindowListener(new WindowAdapter(){
34.
35.public void windowClosing(WindowEvent e){
36.
37.System.exit(0);
38.
39.}
40.
41.});
42.
43.}
44.
45.}
46.
47.abstract class GUIFactory{
48.
49.public abstract Component getButton();
50.
51.public abstract Component getTextField();
52.
53.public abstract Frame getFrame();
54.
55.}
56.
57.class AWTFactory extends GUIFactory{
58.
59.public Component getButton() {
60.
61.return new Button("AWT Button");
62.
63.}
64.
65.public Frame getFrame() {
66.
67.return new Frame("AWT Frame");
68.
69.}
70.
71.public Component getTextField() {
72.
73.return new TextField(20);
74.
75.}
76.
77.
78.
79.}
80.
81.class SwingFactory extends GUIFactory{
82.
83.public Component getButton() {
84.
85.return new JButton("Swing Button");
86.
87.}
88.
89.public Frame getFrame() {
90.
91.return new JFrame("Swing Frame");
92.
93.}
94.
95.public Component getTextField() {
96.
97.return new JTextField(20);
98.
99.}
100.
101.}
3.单例模式(Singleton)改善全局变量和命名空间的冲突,可以说是一种改良了的全局变量。
这种一个类只有一个实例,且提供一个访问全局点的方式,更加灵活的保证了实例的创建和访问约束。
系统中只有一个实例,因此构造方法应该为私有饿汉式:
类加载时直接创建静态实例懒汉式:
第一次需要时才创建一个实例,那么newInstance方法要加同步饿汉式比懒汉式要好,尽管资源利用率要差。
但是不用同步。
[java] viewplaincopy
1.public class TestSingleton {
2.
3.public static void main(String[] args) {
4.
5.
6.
7.}
8.
9.}
10.
11.class ClassA{ //饿汉式
12.
13.private static ClassA i=new ClassA();
14.
15.public static ClassA newInstance(){
16.
17.return i;
18.
19.}
20.
21.private ClassA(){}
22.
23.}
24.
25.class ClassB{ //懒汉式
26.
27.private static ClassB i=null;
28.
29.public static synchronized ClassB newInstance(){
30.
31.if (i==null) i=new ClassB();
32.
33.return i;
34.
35.}
36.
37.private ClassB(){}
38.
39.}
4.建造模式(Builder)将一个对象的内部表象和建造过程分割,一个建造过程可以造出不同表象的对象。
可简化为模版方法模式.
[java] viewplaincopy
1.public class TestBuilder {
2.
3.public static void main(String[] args) {
4.
5.Builder b=new BuilderImpl1();
6.
7.Director d=new Director(b);
8.
9.Product p=d.createProduct();
10.
11.}
12.
13.
14.
15.}
16.
17. interface Builder{
18.
19.void buildPart1();
20.
21.void buildPart2();
22.
23.void buildPart3();
24.
25.Product getProduct();
26.
27.}
28.
29.class BuilderImpl1 implements Builder{
30.
31.
32.
33.public void buildPart1() {
34.
35.System.out.println("create part1");
36.
37. }
38.
39.
40.
41.public void buildPart2() {
42.
43.System.out.println("create part2");
44.
45.}
46.
47.
48.
49.public void buildPart3() {
50.
51.System.out.println("create part3");
52.
53.}
54.
55.
56.
57.public Product getProduct() {
58.
59.return new Product();
60.
61.}
62.
63.
64.
65.}
66.
67.
68.
69.class Director{
70.
71.Builder b;
72.
73.public Director(Builder b){
74.
75.this.b=b;
76.
77.}
78.
79.public Product createProduct(){
80.
81.b.buildPart1(); b.buildPart2();
82.
83.b.buildPart3();
84.
85.return b.getProduct();
86.
87.}
88.
89. }
90.
91.class Product{}
深拷贝:
拷贝本对象引用的对象,有可能会出现循环引用的情况。
可以用串行化解决深拷贝。
写到流里再读出来,这时会是一个对象的深拷贝结果。
浅拷贝:
只拷贝简单属性的值和对象属性的地址5.原型模式(ProtoType)通过一个原型对象来创建一个新对象(克隆)。
Java中要给出Clonable接口的实现,具体类要实现这个接口,并给出clone()方法的实现细节,这就是简单原型模式的应用。
[java] viewplaincopy
1.import java.io.*;
2.
3.public class TestClonealbe {
4.
5.public static void main(String[] args) throws Exception {
6.
7.Father f=new Father();
8.
9.
10.
11.User u1=new User("123456",f);
12.
13.User u2=(User)u1.clone();
14.
15.System.out.println(u1==u2);
16.
17.System.out.println(u1.f==u2.f);
18.
19.}
20.
21.}
22.
23.class User implements Cloneable,Serializable{
24.
25.String password;
26.
27.Father f;
28.
29.public User(String password,Father f){
30.
31.this.password=password;
32.
33.this.f=f;
34.
35.}
36.
37.public Object clone() throws CloneNotSupportedException {
38.
39.//return super.clone();
40.
41.ObjectOutputStream out=null;
42.
43.ObjectInputStream in=null;
44.
45.try {
46.
47.ByteArrayOutputStream bo=new ByteArrayOutputStream();
48.
49.out = new ObjectOutputStream(bo);
50.
51.out.writeObject(this);
52.
53.out.flush();
54.
55.byte[] bs=bo.toByteArray();
56.
57.
58.
59.ByteArrayInputStream bi=new ByteArrayInputStream(bs);
60.
61.in = new ObjectInputStream(bi);
62.
63.Object o=in.readObject();
64.
65.
66.
67.return o;
68.
69.} catch (IOException e) {
70.
71.e.printStackTrace();
72.
73.return null;
74.
75.} catch (ClassNotFoundException e) {
76.
77.e.printStackTrace();
78.
79.return null;
80.
81.}
82.
83.finally{
84.
85.try {
86.
87.out.close();
88.
89.in.close();
90.
91.} catch (IOException e) {
92.
93.e.printStackTrace();
94.
95.}
96.
97.}
98.
99.}
100.
101.}
102.
103.class Father implements Serializable{}
结构模式 如何把简单的类根据某种结构组装为大的系统
6.适配器模式(Adapter)在原类型不做任何改变的情况下,用一个适配器类把一个接口转成另一个接口,扩展了新的接口,灵活且多样的适配一切旧俗。
这种打破旧框框,适配新格局的思想,是面向对象的精髓。
以继承方式实现的类的Adapter模式和以聚合方式实现的对象的Adapter模式,各有千秋,各取所长。
[java] viewplaincopy
1.public class TestAdapter {
2.
3.public static void main(String[] args) {
4.
5.USB mouse=new Mouse();
6.
7.PC pc=new PC();
8.
9.//pc.useMouse(mouse);
10.
11.PS2 adapter=new USB2PS2Adapter(mouse);
12.
13.pc.useMouse(adapter);
14.
15.}
16.
17.}
18.
19.interface PS2{
20.
21.void usePs2();
22.
23.}
24.
25.interface USB{
26.
27.void useUsb();
28.
29.}
30.
31.class Mouse implements USB{
32.
33.public void useUsb(){
34.
35.System.out.println("通过USB接口工作");
36.
37.}
38.
39.}
40.
41.class PC{
42.
43.public void useMouse(PS2 ps2Mouse){
44.
45.ps2Mouse.usePs2();
46.
47.}
48.
49.}
50.
51.class USB2PS2Adapter implements PS2{
52.
53.private USB usb;
54.
55.public USB2PS2Adapter(USB usb) {
56.
57.this.usb = usb;
58.
59.}
60.
61.public void usePs2(){
62.
63.System.out.println("把对usePS2的方法调用转换成对useUSB的方法调用");
64.
65.usb.useUsb();
66.
67.}
68.
69.}
7.组合模式(Composite)把整体和局部的关系用树状结构描述出来,使得客户端把整体对象和局部对象同等看待。
[java] viewplaincopy
1.import java.util.*;
2.
3.public class TestComposite {
4.
5.public static void main(String[] args) {
6.
7.Node n1=new LeafNode(3);
8.
9.Node n2=new LeafNode(4);
10.
11.Node n3=new LeafNode(6);
12.
13.Node n4=new LeafNode(5);
14.
15.Node n5=new LeafNode
(2);
16.
17.Node n6=new LeafNode(9);
18.
19.Node n7=new LeafNode(12);
20.
21.Node n8=new LeafNode(7);
22.
23.Node n9=new LeafNode(8);
24.
25.Node c1=new CompositeNode(n1,n2,n3);
26.
27.Node c4=new CompositeNode(n8,n9);
28.
29.Node c3=new CompositeNode(n5,c4);
30.
31.Node c2=new CompositeNode(n4,c3);
32.
33.Node c5=new CompositeNode(n6,n7);
34.
35.Node root=new CompositeNode(c1,c2,c5);
36.
37.
38.
39.System.out.println(root.getValue());
40.
41.}
104.
105.}
106.
107.abstract class Node{
108.
109.public abstract int getValue();
110.
111.}
112.
113.class LeafNode extends Node{
114.
115.int value;
116.
117.public LeafNode(int value){
118.
119.this.value=value;
120.
12