19State状态模式.docx
《19State状态模式.docx》由会员分享,可在线阅读,更多相关《19State状态模式.docx(15页珍藏版)》请在冰豆网上搜索。
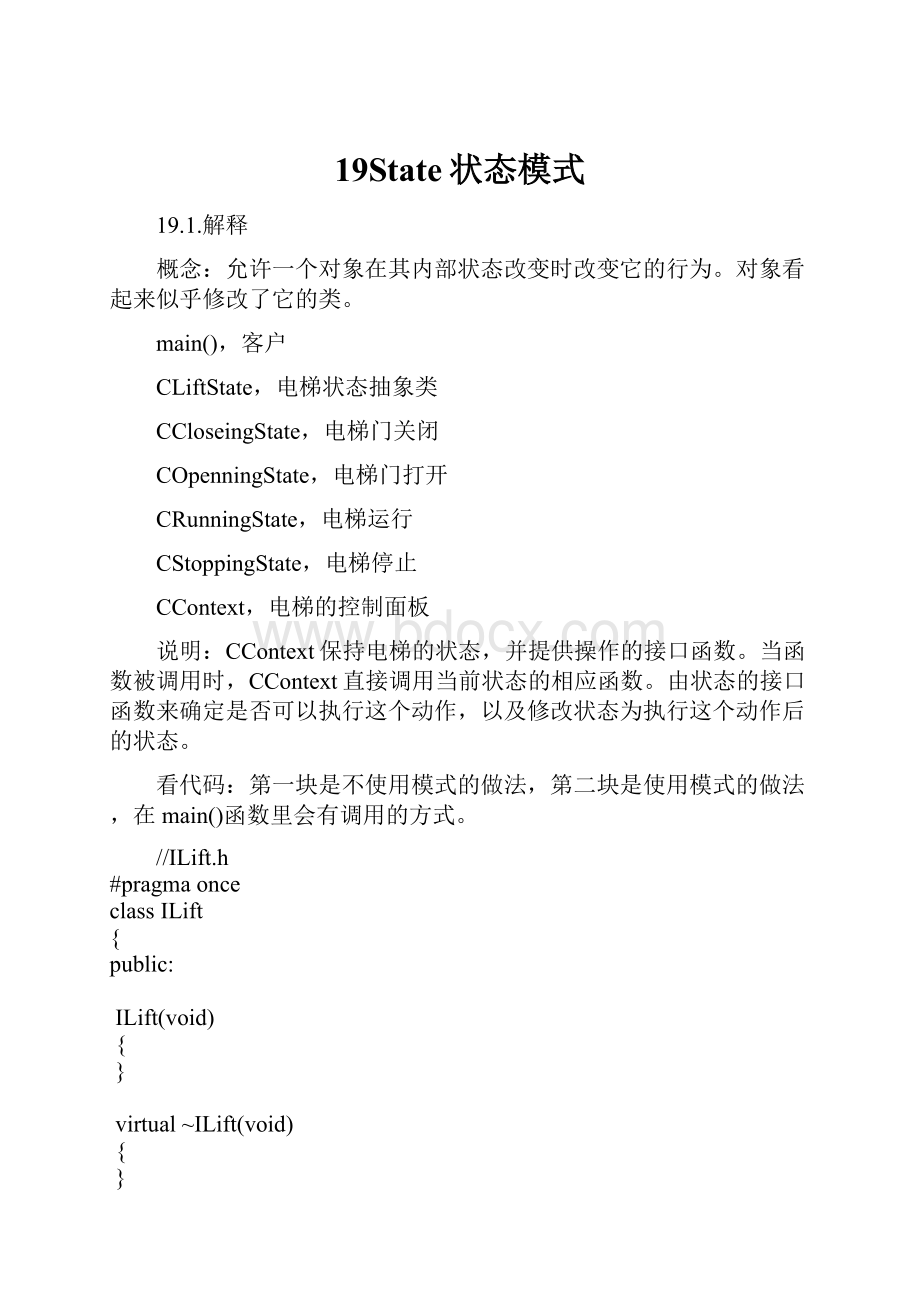
19State状态模式
19.1.解释
概念:
允许一个对象在其内部状态改变时改变它的行为。
对象看起来似乎修改了它的类。
main(),客户
CLiftState,电梯状态抽象类
CCloseingState,电梯门关闭
COpenningState,电梯门打开
CRunningState,电梯运行
CStoppingState,电梯停止
CContext,电梯的控制面板
说明:
CContext保持电梯的状态,并提供操作的接口函数。
当函数被调用时,CContext直接调用当前状态的相应函数。
由状态的接口函数来确定是否可以执行这个动作,以及修改状态为执行这个动作后的状态。
看代码:
第一块是不使用模式的做法,第二块是使用模式的做法,在main()函数里会有调用的方式。
//ILift.h
#pragmaonce
classILift
{
public:
ILift(void)
{
}
virtual~ILift(void)
{
}
staticconstintOPENING_STATE=1;
staticconstintCLOSING_STATE=2;
staticconstintRUNNING_STATE=3;
staticconstintSTOPPING_STATE=4;
virtualvoidSetState(intstate)=0;
virtualvoidOpen()=0;
virtualvoidClose()=0;
virtualvoidRun()=0;
virtualvoidStop()=0;
};
//Lift.h
#pragmaonce
#include"ilift.h"
classCLift:
publicILift
{
public:
CLift(void);
~CLift(void);
voidSetState(intstate);
voidOpen();
voidClose();
voidRun();
voidStop();
private:
intm_state;
voidOpenWithoutLogic();
voidCloseWithoutLogic();
voidRunWithoutLogic();
voidStopWithoutLogic();
};
//Lift.cpp
#include"StdAfx.h"
#include"Lift.h"
#include
usingstd:
:
cout;
usingstd:
:
endl;
CLift:
:
CLift(void)
{
this->m_state=0;
}
CLift:
:
~CLift(void)
{
}
voidCLift:
:
SetState(intstate)
{
this->m_state=state;
}
voidCLift:
:
Open()
{
switch(this->m_state)
{
caseOPENING_STATE:
break;
caseCLOSING_STATE:
this->OpenWithoutLogic();
this->SetState(OPENING_STATE);
break;
caseRUNNING_STATE:
break;
caseSTOPPING_STATE:
this->OpenWithoutLogic();
this->SetState(OPENING_STATE);
break;
}
}
voidCLift:
:
Close()
{
switch(this->m_state)
{
caseOPENING_STATE:
this->CloseWithoutLogic();
this->SetState(CLOSING_STATE);
break;
caseCLOSING_STATE:
break;
caseRUNNING_STATE:
break;
caseSTOPPING_STATE:
break;
}
}
voidCLift:
:
Run()
{
switch(this->m_state)
{
caseOPENING_STATE:
break;
caseCLOSING_STATE:
this->RunWithoutLogic();
this->SetState(RUNNING_STATE);
break;
caseRUNNING_STATE:
break;
caseSTOPPING_STATE:
this->RunWithoutLogic();
this->SetState(RUNNING_STATE);
break;
}
}
voidCLift:
:
Stop()
{
switch(this->m_state)
{
caseOPENING_STATE:
break;
caseCLOSING_STATE:
this->StopWithoutLogic();
this->SetState(CLOSING_STATE);
break;
caseRUNNING_STATE:
this->StopWithoutLogic();
this->SetState(CLOSING_STATE);
break;
caseSTOPPING_STATE:
break;
}
}
voidCLift:
:
OpenWithoutLogic()
{
cout<<"电梯门开启..."<}
voidCLift:
:
CloseWithoutLogic()
{
cout<<"电梯门关闭..."<}
voidCLift:
:
RunWithoutLogic()
{
cout<<"电梯上下跑起来..."<}
voidCLift:
:
StopWithoutLogic()
{
cout<<"电梯停止了..."<}
//LiftState.h
#pragmaonce
classCContext;
classCLiftState
{
public:
CLiftState(void);
virtual~CLiftState(void);
voidSetContext(CContext*pContext);
virtualvoidOpen()=0;
virtualvoidClose()=0;
virtualvoidRun()=0;
virtualvoidStop()=0;
protected:
CContext*m_pContext;
};
//LiftState.cpp
#include"StdAfx.h"
#include"LiftState.h"
CLiftState:
:
CLiftState(void)
{
}
CLiftState:
:
~CLiftState(void)
{
}
voidCLiftState:
:
SetContext(CContext*pContext)
{
m_pContext=pContext;
}
//CloseingState.h
#pragmaonce
#include"liftstate.h"
classCCloseingState:
publicCLiftState
{
public:
CCloseingState(void);
~CCloseingState(void);
voidOpen();
voidClose();
voidRun();
voidStop();
};
//CloseingState.cpp
#include"StdAfx.h"
#include"CloseingState.h"
#include"Context.h"
#include
usingstd:
:
cout;
usingstd:
:
endl;
CCloseingState:
:
CCloseingState(void)
{
}
CCloseingState:
:
~CCloseingState(void)
{
}
voidCCloseingState:
:
Open()
{
this->CLiftState:
:
m_pContext->SetLiftState(CContext:
:
pOpenningState);
this->CLiftState:
:
m_pContext->GetLiftState()->Open();
}
voidCCloseingState:
:
Close()
{
cout<<"电梯门关闭..."<}
voidCCloseingState:
:
Run()
{
this->CLiftState:
:
m_pContext->SetLiftState(CContext:
:
pRunningState);
this->CLiftState:
:
m_pContext->GetLiftState()->Run();
}
voidCCloseingState:
:
Stop()
{
this->CLiftState:
:
m_pContext->SetLiftState(CContext:
:
pStoppingState);
this->CLiftState:
:
m_pContext->GetLiftState()->Stop();
}
//OpenningState.h
#pragmaonce
#include"liftstate.h"
classCOpenningState:
publicCLiftState
{
public:
COpenningState(void);
~COpenningState(void);
voidOpen();
voidClose();
voidRun();
voidStop();
};
//OpenningState.cpp
#include"StdAfx.h"
#include"OpenningState.h"
#include"Context.h"
#include
usingstd:
:
cout;
usingstd:
:
endl;
COpenningState:
:
COpenningState(void)
{
}
COpenningState:
:
~COpenningState(void)
{
}
voidCOpenningState:
:
Open()
{
cout<<"电梯门开启..."<}
voidCOpenningState:
:
Close()
{
this->CLiftState:
:
m_pContext->SetLiftState(CContext:
:
pCloseingState);
this->CLiftState:
:
m_pContext->GetLiftState()->Close();
}
voidCOpenningState:
:
Run()
{
//donothing
}
voidCOpenningState:
:
Stop()
{
//donothing
}
//RunningState.h
#pragmaonce
#include"liftstate.h"
classCRunningState:
publicCLiftState
{
public:
CRunningState(void);
~CRunningState(void);
voidOpen();
voidClose();
voidRun();
voidStop();
};
//RunningState.cpp
#include"StdAfx.h"
#include"RunningState.h"
#include"Context.h"
#include
usingstd:
:
cout;
usingstd:
:
endl;
CRunningState:
:
CRunningState(void)
{
}
CRunningState:
:
~CRunningState(void)
{
}
voidCRunningState:
:
Open()
{
//donothing
}
voidCRunningState:
:
Close()
{
//donothing
}
voidCRunningState:
:
Run()
{
cout<<"电梯上下跑..."<}
voidCRunningState:
:
Stop()
{
this->CLiftState:
:
m_pContext->SetLiftState(CContext:
:
pStoppingState);
this->CLiftState:
:
m_pContext->GetLiftState()->Stop();
}
//StoppingState.h
#pragmaonce
#include"liftstate.h"
classCStoppingState:
publicCLiftState
{
public:
CStoppingState(void);
~CStoppingState(void);
voidOpen();
voidClose();
voidRun();
voidStop();
};
//StoppingState.cpp
#include"StdAfx.h"
#include"StoppingState.h"
#include"Context.h"
#include
usingstd:
:
cout;
usingstd:
:
endl;
CStoppingState:
:
CStoppingState(void)
{
}
CStoppingState:
:
~CStoppingState(void)
{
}
voidCStoppingState:
:
Open()
{
this->CLiftState:
:
m_pContext->SetLiftState(CContext:
:
pOpenningState);
this->CLiftState:
:
m_pContext->GetLiftState()->Open();
}
voidCStoppingState:
:
Close()
{
//donothing
}
voidCStoppingState:
:
Run()
{
this->CLiftState:
:
m_pContext->SetLiftState(CContext:
:
pRunningState);
this->CLiftState:
:
m_pContext->GetLiftState()->Run();
}
voidCStoppingState:
:
Stop()
{
cout<<"电梯停止了..."<}
//Contex.h
#pragmaonce
#include"LiftState.h"
#include"OpenningState.h"
#include"CloseingState.h"
#include"RunningState.h"
#include"StoppingState.h"
classCContext
{
public:
CContext(void);
~CContext(void);
staticCOpenningState*pOpenningState;
staticCCloseingState*pCloseingState;
staticCRunningState*pRunningState;
staticCStoppingState*pStoppingState;
CLiftState*GetLiftState();
voidSetLiftState(CLiftState*pLiftState);
voidOpen();
voidClose();
voidRun();
voidStop();
private:
CLiftState*m_pLiftState;
};
//Context.cpp
#include"StdAfx.h"
#include"Context.h"
COpenningState*CContext:
:
pOpenningState=NULL;
CCloseingState*CContext:
:
pCloseingState=NULL;
CRunningState*CContext:
:
pRunningState=NULL;
CStoppingState*CContext:
:
pStoppingState=NULL;
CContext:
:
CContext(void)
{
m_pLiftState=NULL;
pOpenningState=newCOpenningState();
pCloseingState=newCCloseingState();
pRunningState=newCRunningState();
pStoppingState=newCStoppingState();
}
CContext:
:
~CContext(void)
{
deletepOpenningState;
pOpenningState=NULL;
deletepCloseingState;
pCloseingState=NULL;
deletepRunningState;
pRunningState=NULL;
deletepStoppingState;
pStoppingState=NULL;
}
CLiftState*CContext:
:
GetLiftState()
{
returnm_pLiftState;
}
voidCContext:
:
SetLiftState(CLiftState*pLiftState)
{
this->m_pLiftState=pLiftState;
this->m_pLiftState->SetContext(this);
}
voidCContext:
:
Open()
{
this->m_pLiftState->Open();
}
voidCContext:
:
Close()
{
this->m_pLiftState->Close();
}
voidCContext:
:
Run()
{
this->m_pLiftState->Run();
}
voidCContext:
:
Stop()
{
this->m_pLiftState->Stop();
}
//State.cpp:
定义控制台应用程序的入口点。
#include"stdafx.h"
#include"ILift.h"
#include"Lift.h"
#include"Context.h"
#include"OpenningState.h"
#include"CloseingState.h"
#include"RunningState.h"
#include"StoppingState.h"
#include
usingstd:
:
cout;
usingstd:
:
endl;
voidDoIt()
{
//ILift.h,Lift.h,Lift.cpp
ILift*pLift=newCLift();
pLift->SetState(ILift:
:
STOPPING_STATE);//电梯的初始条件是停止状态。
pLift->Open();//首先是电梯门开启,人进去
pLift->Close();//然后电梯门关闭
pLift->Run();//再然后,电梯跑起来,向上或者向下
pLift->Stop();//最后到达目的地,电梯停下来
deletepLift;
}
voidDoNew()
{
//LiftState.h,LiftState.cpp,OpenningState.h,CloseingState.h,RunningState.h,StoppingState.h
//Context.h,Context.cpp
CContextcontext;
CCloseingStatecloseingState;
context.SetLiftState(&closeingState);
context.Close();
context.Open();
context.Run();
context.Stop();
}
int_tmain(intargc,_TCHAR*argv[])
{
cout<<"----------使用模式之前----------"<DoIt();
cout<<"----------使用模式之后----------"<DoNew();
_CrtSetDbgFlag(_CRTDBG_LEAK_CHECK_DF|_CRTDBG_ALLOC_MEM_DF);
_CrtDumpMemoryLeaks();
return0;
}
状态模式也是行为型模式。
写了好多天了,才发现如何在页面上插入代码。
这样方便多了,看起来也清楚多了。
博客园也许需要开个入门贴子,免得新来博客园的童鞋都不大会用。
这几天还在学习数据结构中的排序,入排序入手来学习数据结构还是比较容易的。
上学的时候只顾着考试了,该学的也都学了,但考完试就全忘掉,已经成为了一种习惯。
现在再补回来。
鼓励自己加油!
一定要做一个学习型的程序员。