计算机图形学大作业.docx
《计算机图形学大作业.docx》由会员分享,可在线阅读,更多相关《计算机图形学大作业.docx(24页珍藏版)》请在冰豆网上搜索。
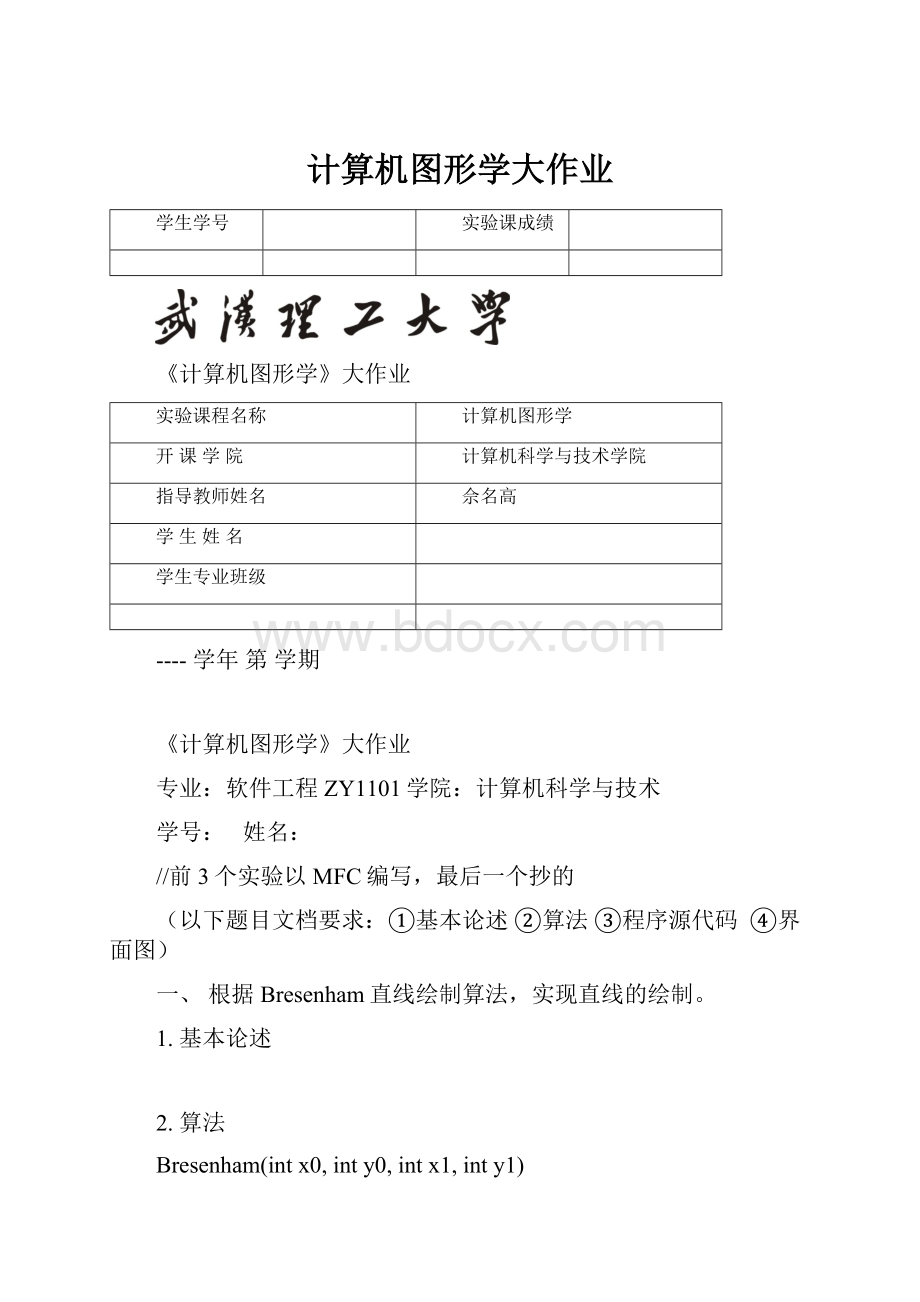
计算机图形学大作业
学生学号
实验课成绩
《计算机图形学》大作业
实验课程名称
计算机图形学
开课学院
计算机科学与技术学院
指导教师姓名
佘名高
学生姓名
学生专业班级
----学年第学期
《计算机图形学》大作业
专业:
软件工程ZY1101学院:
计算机科学与技术
学号:
姓名:
//前3个实验以MFC编写,最后一个抄的
(以下题目文档要求:
①基本论述②算法③程序源代码④界面图)
一、根据Bresenham直线绘制算法,实现直线的绘制。
1.基本论述
2.算法
Bresenham(intx0,inty0,intx1,inty1)
{
intdx,dy,e,i,x,y;
dx=x1-x0;
dy=y1-y0;
e=2*dy-dx;
x=x0;
y=y0;
for(i=0;i<=dx;i++)
{
CDC*pDC=this->GetDC();
pDC->SetPixel(x,y,RGB(0,0,0));
if(e>=0)
{
y++;
e=e-2*dx;
}
else
x++;
e=e+2*dy;
}
}
3.程序源代码
//LINE2View.cpp:
implementationoftheCLINE2Viewclass
//
#include"math.h"
#include"stdafx.h"
#include"LINE2.h"
#include"LINE2Doc.h"
#include"LINE2View.h"
#ifdef_DEBUG
#definenewDEBUG_NEW
#undefTHIS_FILE
staticcharTHIS_FILE[]=__FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
//CLINE2View
IMPLEMENT_DYNCREATE(CLINE2View,CView)
BEGIN_MESSAGE_MAP(CLINE2View,CView)
//{{AFX_MSG_MAP(CLINE2View)
//NOTE-theClassWizardwilladdandremovemappingmacroshere.
//DONOTEDITwhatyouseeintheseblocksofgeneratedcode!
//}}AFX_MSG_MAP
//Standardprintingcommands
ON_COMMAND(ID_FILE_PRINT,CView:
:
OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_DIRECT,CView:
:
OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_PREVIEW,CView:
:
OnFilePrintPreview)
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
//CLINE2Viewconstruction/destruction
CLINE2View:
:
CLINE2View()
{
//TODO:
addconstructioncodehere
}
CLINE2View:
:
~CLINE2View()
{
}
BOOLCLINE2View:
:
PreCreateWindow(CREATESTRUCT&cs)
{
//TODO:
ModifytheWindowclassorstylesherebymodifying
//theCREATESTRUCTcs
returnCView:
:
PreCreateWindow(cs);
}
/////////////////////////////////////////////////////////////////////////////
//CLINE2Viewdrawing
voidCLINE2View:
:
OnDraw(CDC*pDC)
{Bresenham(100,100,800,180);
CLINE2Doc*pDoc=GetDocument();
ASSERT_VALID(pDoc);
//TODO:
adddrawcodefornativedatahere
}
/////////////////////////////////////////////////////////////////////////////
//CLINE2Viewprinting
BOOLCLINE2View:
:
OnPreparePrinting(CPrintInfo*pInfo)
{
//defaultpreparation
returnDoPreparePrinting(pInfo);
}
voidCLINE2View:
:
OnBeginPrinting(CDC*/*pDC*/,CPrintInfo*/*pInfo*/)
{
//TODO:
addextrainitializationbeforeprinting
}
voidCLINE2View:
:
OnEndPrinting(CDC*/*pDC*/,CPrintInfo*/*pInfo*/)
{
//TODO:
addcleanupafterprinting
}
/////////////////////////////////////////////////////////////////////////////
//CLINE2Viewdiagnostics
#ifdef_DEBUG
voidCLINE2View:
:
AssertValid()const
{
CView:
:
AssertValid();
}
voidCLINE2View:
:
Dump(CDumpContext&dc)const
{
CView:
:
Dump(dc);
}
CLINE2Doc*CLINE2View:
:
GetDocument()//non-debugversionisinline
{
ASSERT(m_pDocument->IsKindOf(RUNTIME_CLASS(CLINE2Doc)));
return(CLINE2Doc*)m_pDocument;
}
#endif//_DEBUG
/////////////////////////////////////////////////////////////////////////////
//CLINE2Viewmessagehandlers
voidCLINE2View:
:
Bresenham(intx0,inty0,intx1,inty1){
intdx,dy,e,i,x,y;
dx=x1-x0;
dy=y1-y0;
e=2*dy-dx;
x=x0;
y=y0;
for(i=0;i<=dx;i++)
{
CDC*pDC=this->GetDC();
pDC->SetPixel(x,y,RGB(0,0,0));
if(e>=0)
{
y++;
e=e-2*dx;
}
else
x++;
e=e+2*dy;
}
}
4.程序运行截图
二、用C语言编写:
画y=sin(x)的图形(要求画出[-2π,2π]的图形及笛卡尔坐标)
1.基本论述
2.算法
drawSin(intx,inty,intA)
{
CDC*pDC=this->GetDC();
inti=0;
pDC->MoveTo(x-300,y);
pDC->LineTo(x+300,y);
pDC->LineTo(x+290,y-10);
pDC->MoveTo(x+300,y);
pDC->LineTo(x+290,y+10);
pDC->MoveTo(x,y+200);
pDC->LineTo(x,y-200);
pDC->LineTo(x-10,y-190);
pDC->MoveTo(x,y-200);
pDC->LineTo(x+10,y-190);
pDC->MoveTo(-100000,0);
for(i=-314/2;i<(314/2);i++)
{
pDC->SetPixel(x+i,(int)A*sin((double)i/25)+y,RGB(0,0,0));
pDC->LineTo(x+i,(int)A*sin((double)i/25)+y);
}
}
3.程序源代码
//sin2View.cpp:
implementationoftheCSin2Viewclass
//
#include"stdafx.h"
#include"sin2.h"
#include"math.h"
#include"sin2Doc.h"
#include"sin2View.h"
#ifdef_DEBUG
#definenewDEBUG_NEW
#undefTHIS_FILE
staticcharTHIS_FILE[]=__FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
//CSin2View
IMPLEMENT_DYNCREATE(CSin2View,CView)
BEGIN_MESSAGE_MAP(CSin2View,CView)
//{{AFX_MSG_MAP(CSin2View)
//NOTE-theClassWizardwilladdandremovemappingmacroshere.
//DONOTEDITwhatyouseeintheseblocksofgeneratedcode!
//}}AFX_MSG_MAP
//Standardprintingcommands
ON_COMMAND(ID_FILE_PRINT,CView:
:
OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_DIRECT,CView:
:
OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_PREVIEW,CView:
:
OnFilePrintPreview)
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
//CSin2Viewconstruction/destruction
CSin2View:
:
CSin2View()
{
//TODO:
addconstructioncodehere
}
CSin2View:
:
~CSin2View()
{
}
BOOLCSin2View:
:
PreCreateWindow(CREATESTRUCT&cs)
{
//TODO:
ModifytheWindowclassorstylesherebymodifying
//theCREATESTRUCTcs
returnCView:
:
PreCreateWindow(cs);
}
/////////////////////////////////////////////////////////////////////////////
//CSin2Viewdrawing
voidCSin2View:
:
OnDraw(CDC*pDC)
{drawSin(300,200,100);
CSin2Doc*pDoc=GetDocument();
ASSERT_VALID(pDoc);
//TODO:
adddrawcodefornativedatahere
}
/////////////////////////////////////////////////////////////////////////////
//CSin2Viewprinting
BOOLCSin2View:
:
OnPreparePrinting(CPrintInfo*pInfo)
{
//defaultpreparation
returnDoPreparePrinting(pInfo);
}
voidCSin2View:
:
OnBeginPrinting(CDC*/*pDC*/,CPrintInfo*/*pInfo*/)
{
//TODO:
addextrainitializationbeforeprinting
}
voidCSin2View:
:
OnEndPrinting(CDC*/*pDC*/,CPrintInfo*/*pInfo*/)
{
//TODO:
addcleanupafterprinting
}
/////////////////////////////////////////////////////////////////////////////
//CSin2Viewdiagnostics
#ifdef_DEBUG
voidCSin2View:
:
AssertValid()const
{
CView:
:
AssertValid();
}
voidCSin2View:
:
Dump(CDumpContext&dc)const
{
CView:
:
Dump(dc);
}
CSin2Doc*CSin2View:
:
GetDocument()//non-debugversionisinline
{
ASSERT(m_pDocument->IsKindOf(RUNTIME_CLASS(CSin2Doc)));
return(CSin2Doc*)m_pDocument;
}
#endif//_DEBUG
/////////////////////////////////////////////////////////////////////////////
//CSin2Viewmessagehandlers
voidCSin2View:
:
drawSin(intx,inty,intA)
{
CDC*pDC=this->GetDC();
inti=0;
pDC->MoveTo(x-300,y);
pDC->LineTo(x+300,y);
pDC->LineTo(x+290,y-10);
pDC->MoveTo(x+300,y);
pDC->LineTo(x+290,y+10);
pDC->MoveTo(x,y+200);
pDC->LineTo(x,y-200);
pDC->LineTo(x-10,y-190);
pDC->MoveTo(x,y-200);
pDC->LineTo(x+10,y-190);
pDC->MoveTo(-100000,0);
for(i=-314/2;i<(314/2);i++)
{
pDC->SetPixel(x+i,(int)A*sin((double)i/25)+y,RGB(0,0,0));
pDC->LineTo(x+i,(int)A*sin((double)i/25)+y);
}
}
4.程序运行截图
三、用C语言编写一个小圆沿着大圆运动的程序。
1.基本论述
2.算法
for(i=0;i<=1800;i++)
{pDC->Ellipse(220,340,420,140);pDC->Ellipse(320+100*cos(x)+20,240+100*sin(x)+20,320+100*cos(x)-20,240+100*sin(x)-20);
x=x+3.1415926/180;
Sleep(200);
3.程序源代码
//circlesView.cpp:
implementationoftheCCirclesViewclass
//
#include"stdafx.h"
#include"circles.h"
#include"math.h"
#include"circlesDoc.h"
#include"circlesView.h"
#ifdef_DEBUG
#definenewDEBUG_NEW
#undefTHIS_FILE
staticcharTHIS_FILE[]=__FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
//CCirclesView
IMPLEMENT_DYNCREATE(CCirclesView,CView)
BEGIN_MESSAGE_MAP(CCirclesView,CView)
//{{AFX_MSG_MAP(CCirclesView)
//NOTE-theClassWizardwilladdandremovemappingmacroshere.
//DONOTEDITwhatyouseeintheseblocksofgeneratedcode!
//}}AFX_MSG_MAP
//Standardprintingcommands
ON_COMMAND(ID_FILE_PRINT,CView:
:
OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_DIRECT,CView:
:
OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_PREVIEW,CView:
:
OnFilePrintPreview)
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
//CCirclesViewconstruction/destruction
CCirclesView:
:
CCirclesView()
{
//TODO:
addconstructioncodehere
}
CCirclesView:
:
~CCirclesView()
{
}
BOOLCCirclesView:
:
PreCreateWindow(CREATESTRUCT&cs)
{
//TODO:
ModifytheWindowclassorstylesherebymodifying
//theCREATESTRUCTcs
returnCView:
:
PreCreateWindow(cs);
}
/////////////////////////////////////////////////////////////////////////////
//CCirclesViewdrawing
voidCCirclesView:
:
OnDraw(CDC*pDC)
{
inti;
floatx;
x=0;
this->GetDC();
for(i=0;i<=1800;i++)
{pDC->Ellipse(220,340,420,140);
pDC->Ellipse(320+100*cos(x)+20,240+100*sin(x)+20,320+100*cos(x)-20,240+100*sin(x)-20);
x=x+3.1415926/180;
Sleep(200);
}
CCirclesDoc*pDoc=GetDocument();
ASSERT_VALID(pDoc);
//TODO:
adddrawcodefornativedatahere
}
/////////////////////////////////////////////////////////////////////////////
//CCirclesViewprinting
BOOLCCirclesView:
:
OnPreparePrinting(CPrintInfo*pInfo)
{
//defaultpreparation
returnDoPreparePrinting(pInfo);
}
voidCCirclesView:
:
OnBeginPrinting(CDC*/*pDC*/,CPrintInfo*/*pInfo*/)
{
//TODO:
addextrainitializationbeforeprinting
}
voidCCirclesView:
:
OnEndPrinting(CDC*/*pDC*/,CPrintInfo*/*pInfo*/)
{
//TODO:
addcleanupafterprinting
}
/////////////////////////////////////////////////////////////////////////////
//CCirclesViewdiagnostics
#ifdef_DEBUG
voidCCirclesView:
:
AssertValid()const
{
CView:
:
AssertValid();
}
voidCCirclesView:
:
Dump(CDumpContext&dc)const
{
CView:
:
Dump(dc);
}
CCirclesDoc*CCirclesView:
:
GetDocument()//non-debugversionisinline
{
ASSERT(m_pDocument->IsKindOf(RU