数据结构课程设计.docx
《数据结构课程设计.docx》由会员分享,可在线阅读,更多相关《数据结构课程设计.docx(57页珍藏版)》请在冰豆网上搜索。
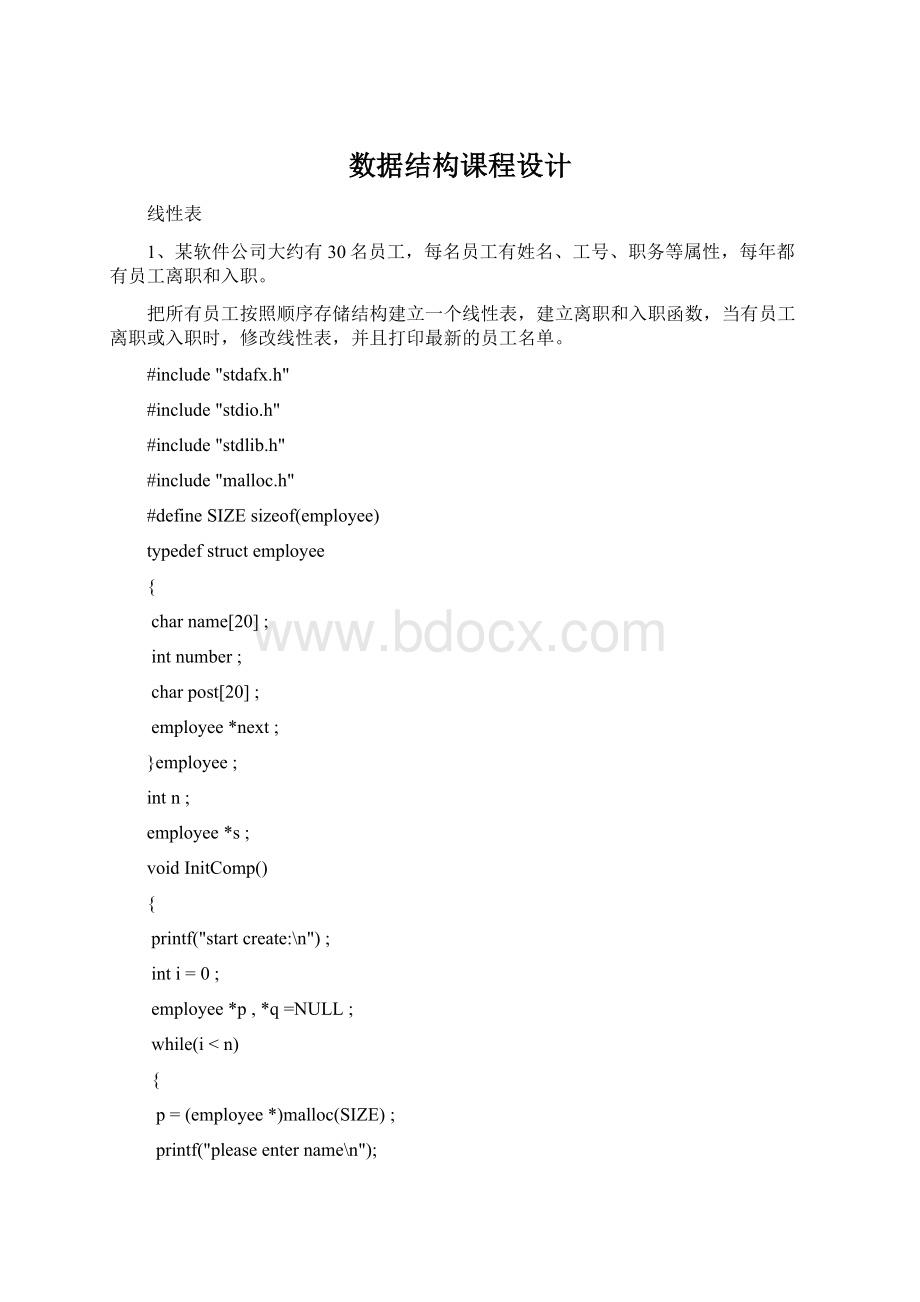
数据结构课程设计
线性表
1、某软件公司大约有30名员工,每名员工有姓名、工号、职务等属性,每年都有员工离职和入职。
把所有员工按照顺序存储结构建立一个线性表,建立离职和入职函数,当有员工离职或入职时,修改线性表,并且打印最新的员工名单。
#include"stdafx.h"
#include"stdio.h"
#include"stdlib.h"
#include"malloc.h"
#defineSIZEsizeof(employee)
typedefstructemployee
{
charname[20];
intnumber;
charpost[20];
employee*next;
}employee;
intn;
employee*s;
voidInitComp()
{
printf("startcreate:
\n");
inti=0;
employee*p,*q=NULL;
while(i{
p=(employee*)malloc(SIZE);
printf("pleaseentername\n");
scanf_s("%s",&(p->name),20);
printf("pleaseenternumber\n");
scanf_s("%d",&(p->number));
printf("pleaseenterpost\n");
scanf_s("%s",&(p->post),20);
p->next=NULL;
i++;
if(i==1)
{
s=p;
q=p;
}
else{
q->next=p;
q=q->next;
}
}
}
voidEmpInsert()
{
employee*p,*q=s;
while(q->next!
=NULL)
q=q->next;
p=(employee*)malloc(SIZE);
printf("pleaseentername\n");
scanf_s("%s",&p->name,20);
printf("pleaseenternumber\n");
scanf_s("%d",&p->number);
printf("pleaseenterpost\n");
scanf_s("%s",&p->post,20);
q->next=p;
p->next=NULL;
n++;
}
voidEmpDelete(intnum)
{
employee*p=s,*q=s;
inti=0,j=0;
while(ji=p->number;
if(i==num){
if(p==s){
s=s->next;
}
else{
q->next=p->next;
}
n--;
return;
}
else{
q=p;
p=p->next;
j++;
}
}
printf("numbernotfound\n");
}
voidEmpPrint()
{
employee*p=s;
printf("thelistofemployees\n");
while(p!
=NULL)
{
printf("%s\t%d\t%s\n",p->name,p->number,p->post);
p=p->next;
}
}
int_tmain(intargc,_TCHAR*argv[])
{
intl,m;
printf("createlist,pleaseenterthenumberoftheemployee\n");
scanf_s("%d",&n);
InitComp();
EmpPrint();
while
(1)
{
printf("enternumbertochooseaction:
1forindert,2fordelete\n");
scanf_s("%d",&l);
switch(l)
{
case1:
EmpInsert();
EmpPrint();
break;
case2:
printf("pleaseenterthenumberoftheemployeeyoudelete\n");
scanf_s("%d",&m);
EmpDelete(m);
EmpPrint();
break;
default:
EmpPrint();
}
}
system("pause");
return0;
}
2、约瑟夫(Josephus)环问题:
编号为1,2,3,…,n的n个人按顺时针方向围坐一圈,每人持有一个密码(正整数)。
一开始任选一个正整数作为报数的上限值m,从第一个人开始按顺时针方向自1开始顺序报数,报到m时停止。
报m的人出列,将他的密码作为新的m值,从他在顺时针方向上的下一人开始重新从1报数,如此下去,直到所有人全部出列为止。
建立n个人的单循环链表存储结构,运行结束后,输出依次出队的人的序号。
#include"stdafx.h"
#include"stdio.h"
#include"stdlib.h"
structperson
{
intnum;
intcode;
person*next;
};
person*h;
voidCreaCircle()
{
intn,i;
person*p,*q=NULL,*r=NULL;
printf("pleaseenterthrnemberofpeople:
\n");
scanf_s("%d",&n);
for(i=1;i{
p=(person*)malloc(sizeof(person));
p->num=i;
printf("pleaseenterthecodeofthe%dperson:
\n",i);
scanf_s("%d",&p->code);
p->next=NULL;
if(i==1)
{
h=p;
q=p;
}
q->next=p;
q=p;
}
q->next=h;
}
voidRunGame()
{
intm,i;
person*r,*t=h;
printf("pleaseenterthefirstcode:
\n");
scanf_s("%d",&m);
while(t->next!
=t)
{
for(i=1;it=t->next;
r=t->next;
m=r->code;
t->next=r->next;
printf("the%dpersoniskicked\n",r->num);
}
}
intmain(){
CreaCircle();
RunGame();
system("pause");
return0;
}
栈和队列
3、某商场有一个100个车位的停车场,当车位未满时,等待的车辆可以进入并计时;当车位已满时,必须有车辆离开,等待的车辆才能进入;当车辆离开时计算停留的的时间,并且按照每小时1元收费。
汽车的输入信息格式可以是(进入/离开,车牌号,进入/离开时间),要求可以随时显示停车场内的车辆信息以及收费历史记录。
#include
#include"time.h"
#include"stdlib.h"
#include
#include"stdio.h"
usingnamespacestd;
#defineLIST_INIT_SIZE10
#definePRICE1
typedefstructCar
{
intcarnumber;
intmoney;
time_tentertime;
time_tleavetime;
}Car;
typedefstructParkList{
Car*head;
intlength;
intlistsize;
}ParkList;
typedefstructWaitNode{
CarWaitCar;
WaitNode*next;
}WaitNode,*WaitList;
typedefstructLeaveNode{
CarLeaveCar;
LeaveNode*next;
}LeaveNode,*LeaveList;
intinit_LeaveList(LeaveList&L){
L=(LeaveList)malloc(sizeof(LeaveNode));
L->next=NULL;
}
intinit_WaitList(WaitList&WL,Carwc){
WL=(WaitList)malloc(sizeof(WaitNode));
WL->WaitCar=wc;
WL->next=NULL;
}
voidPut_LeaveList(LeaveList&L,Carlc){
LeaveListp=L;
if(p==NULL){
return;
}
while(p->next){
p=p->next;
}
LeaveListq=(LeaveList)malloc(sizeof(LeaveNode));
q->LeaveCar=lc;
p->next=q;
q->next=NULL;
q->LeaveCar.money=(q->LeaveCar.leavetime-q->LeaveCar.entertime)*PRICE;
}
voidPutelem_WaitList(WaitList&WL,Carwc){
WaitListp=WL;
if(p==NULL){
init_WaitList(WL,wc);
return;
}
while(p->next){
p=p->next;
}
WaitListq=(WaitList)malloc(sizeof(WaitNode));
q->WaitCar=wc;
p->next=q;
q->next=NULL;
}
voiddelete_WaitList(WaitList&WL,Car&c){
if(WL==NULL){
return;
}
WaitListp=WL;
c=p->WaitCar;
if(WL->next==NULL){
WL=NULL;
}
else{
WL=WL->next;
}
free(p);
}
CarCreateCar(intcarnum){
Car*pc=(Car*)malloc(sizeof(Car));
pc->carnumber=carnum;
pc->entertime=time(NULL);
return*pc;
}
intinit_Parklist(ParkList&p)
{
p.head=(Car*)malloc(LIST_INIT_SIZE*sizeof(Car));
if(!
p.head)return0;
p.length=0;
p.listsize=LIST_INIT_SIZE;
return1;
}
intput_ParkList(ParkList&p,WaitList&WL,Carc)
{
if(p.length==p.listsize){
if(WL==NULL){
init_WaitList(WL,c);
}
elsePutelem_WaitList(WL,c);
return0;
}
Car*pc=p.head;
pc[p.length]=c;
p.length+=1;
return1;
}
intinsert_ParkList(ParkList&p,WaitList&WL,inti)
{
Carc;
delete_WaitList(WL,c);
if(i<0||i>=p.length){return0;}
Car*pc=p.head;
for(intj=p.length-1;j>i-1;j--)
{
pc[j+1]=pc[j];
}
pc[i]=c;
p.length+=1;
return1;
}
intdelete_ParkList(ParkList&p,LeaveList&L,inti)
{
if(i>=0&&i
{
Car*pc=p.head;
pc[i].leavetime=time(NULL);
Put_LeaveList(L,pc[i]);
for(intj=i;j
{
pc[j]=pc[j+1];
}
p.length-=1;
return1;
}
elsereturn0;
}
voidshowParkList(ParkListp)
{
if(p.head!
=NULL)
{
Car*pc=p.head;
printf("ParkList:
\n");
for(intj=0;j
{
printf("%d%s\n",pc[j].carnumber,ctime(&pc[j].entertime));
}
printf("\n");
}
}
voidshowWaitList(WaitListWL){
WaitListp=WL;
printf("WaitList:
\n");
while(p){
printf("%d%s\n",p->WaitCar.carnumber,ctime(&(p->WaitCar.entertime)));
p=p->next;
}
}
voidshowLeaveList(LeaveListL){
LeaveListp=L->next;
printf("LeaveList:
\n");
while(p){
printf("%d%s\n",p->LeaveCar.carnumber,
ctime(&(p->LeaveCar.entertime)));
printf("%s%d\n",ctime(&(p->LeaveCar.leavetime)),p->LeaveCar.money);
p=p->next;
}
}
intmain()
{
ParkListp;
LeaveListL;
WaitListWL=NULL;
init_LeaveList(L);
init_Parklist(p);
srand(time(NULL));
Carc;
inti=1,n=0;
intdic=0;
intindex=0;
inttime=0;
while(i<=100){
dic=rand()%2;
switch(dic){
case1:
c=CreateCar(1000+i);
put_ParkList(p,WL,c);
break;
case0:
if(p.length==0){
break;
}
else{
index=rand()%p.length;
delete_ParkList(p,L,index);
if(WL!
=NULL){
insert_ParkList(p,WL,index);
}
break;
}
default:
break;
}
i++;
}
showParkList(p);
showWaitList(WL);
showLeaveList(L);
}
4、某银行营业厅共有6个营业窗口,设有排队系统广播叫号,该银行的业务分为公积金、银行卡、理财卡等三种。
公积金业务指定1号窗口,银行卡业务指定2、3、4号窗口,理财卡业务指定5、6号窗口。
但如果5、6号窗口全忙,而2、3、4号窗口有空闲时,理财卡业务也可以在空闲的2、3、4号窗口之一办理。
客户领号、业务完成可以作为输入信息,要求可以随时显示6个营业窗口的状态。
#include"stdafx.h"
#include"stdio.h"
#include"stdlib.h"
typedefstructCost
{
intnum;
Cost*next;
}Cost,*CostList;
voidInitCL(CostList&CL,intnumber)
{
CL=(CostList)malloc(sizeof(Cost));
CL->num=number;
CL->next=NULL;
}
voidPutCL(CostList&CL,intnumber)
{
CostListp=CL,q;
if(CL==NULL)
InitCL(CL,number);
else
{
while(p->next)
p=p->next;
q=(CostList)malloc(sizeof(Cost));
q->num=number;
p->next=q;
q->next=NULL;
}
}
voidPopCL(CostList&CL,int&number)
{
CostListp=CL;
if(CL==NULL)
number=0;
else
{
number=CL->num;
CL=CL->next;
free(p);
}
}
voidShowCL(CostList&CL)
{
CostListp=CL;
while(p)
{
printf("%d",p->num);
p=p->next;
}
printf("\n");
}
int_tmain(intargc,_TCHAR*argv[])
{
inta[6]={0},n,costnum=0,i=0,j=0,m;
CostListCL1=NULL,CL2=NULL,CL3=NULL;
while
(1)
{
printf("请选择业务种类\n1.公积金业务\n2.银行卡业务\n3.理财卡业务\n4.业务办理完成\n5.显示当前窗口营业状态\n6.显示当前排队情况\n");
scanf_s("%d",&n);
switch(n)
{
case1:
i++;
printf("您的序号为:
%d\n",i);
if(CL1==NULL)
InitCL(CL1,i);
else
PutCL(CL1,i);
break;
case2:
i++;
printf("您的序号为:
%d\n",i);
if(CL2==NULL)
InitCL(CL2,i);
else
PutCL(CL2,i);
break;
case3:
i++;
printf("您的序号为:
%d\n",i);
if(CL3==NULL)
InitCL(CL3,i);
else
PutCL(CL3,i);
break;
case4:
printf("正在营业的窗口:
\n");
for(j=0;j<6;j++)
{
if(a[j]!
=0)
printf("%d",j+1);
}
printf("\n");
printf("请选择业务办理完成的窗口号:
\n");
scanf_s("%d",&m);
a[m-1]=0;
break;
case5:
printf("窗口营业情况:
\n");
for(j=0;j<6;j++)
{
if(a[j]!
=0)
printf("%d号窗口正在为%d号客户服务\n",j+1,a[j]);
}
break;
case6:
printf("办理公积金业务的客户有:
\n");
ShowCL(CL1);
printf("办理银行卡业务的客户有:
\n");
ShowCL(CL2);
printf("办理理财卡业务的客户有:
\n");
ShowCL(CL3);
}
if(a[0]==0)
{
PopCL(CL1,costnum);
a[0]=costnum;
}
if(a[4]==0)
{
PopCL(CL3,costnum);
a[4]=costnum;
}
if(a[5]==0)
{
PopCL(CL3,costnum);
a[5]=costnum;
}
if(a[1]==0)
{
if(CL2!
=NULL)