C程序设计语言英文版已改格式汇总Word文档下载推荐.docx
《C程序设计语言英文版已改格式汇总Word文档下载推荐.docx》由会员分享,可在线阅读,更多相关《C程序设计语言英文版已改格式汇总Word文档下载推荐.docx(145页珍藏版)》请在冰豆网上搜索。
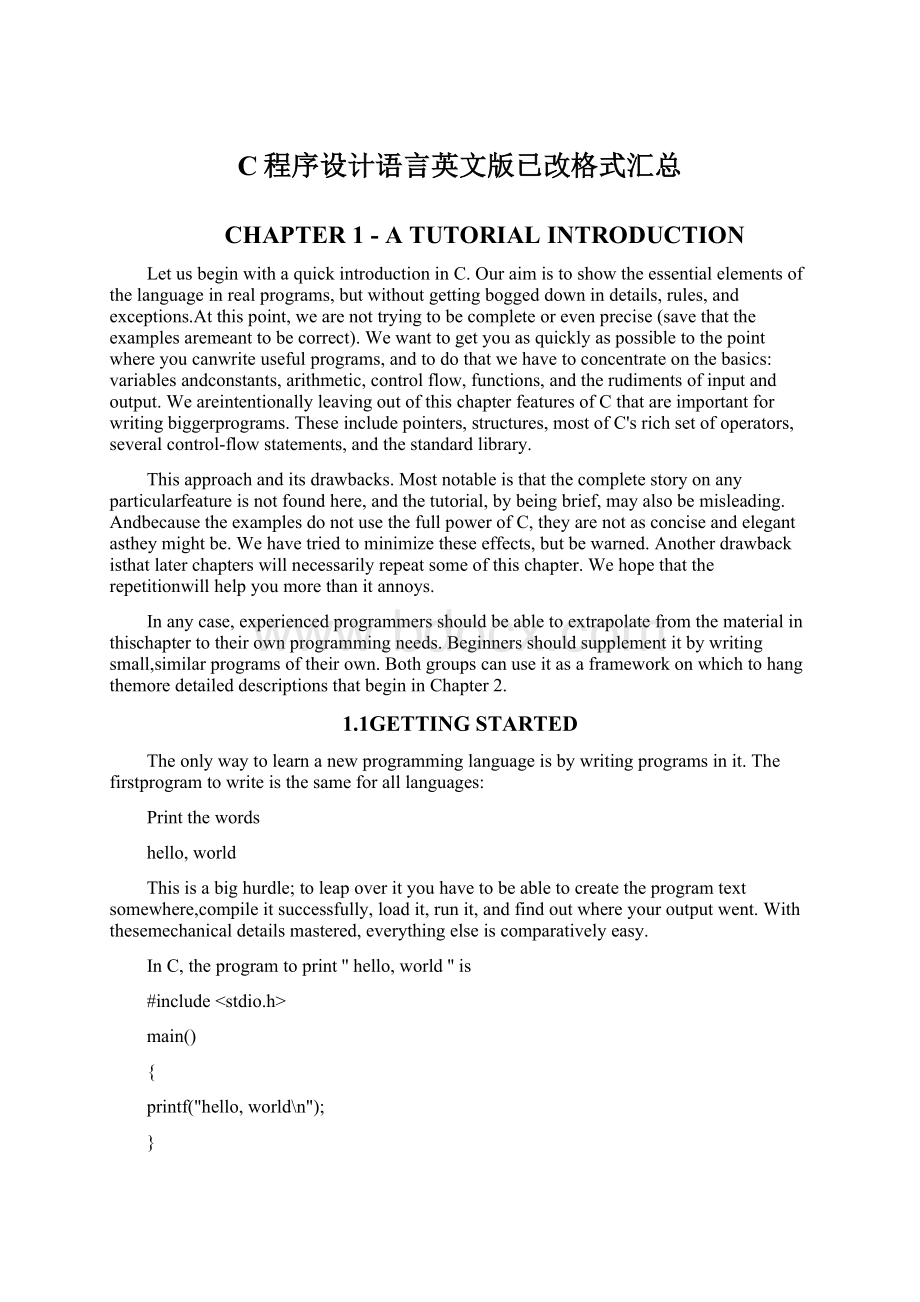
toleapoverityouhavetobeabletocreatetheprogramtextsomewhere,compileitsuccessfully,loadit,runit,andfindoutwhereyouroutputwent.Withthesemechanicaldetailsmastered,everythingelseiscomparativelyeasy.
InC,theprogramtoprint'
'
hello,world'
is
#include<
stdio.h>
main()
{
printf("
hello,world\n"
);
}
Justhowtorunthisprogramdependsonthesystemyouareusing.Asaspecificexample,ontheUNIXoperatingsystemyoumustcreatetheprograminafilewhosenameendsin'
.c'
suchashello.c,thencompileitwiththecommand
cchello.c
Ifyouhaven'
tbotchedanything,suchasomittingacharacterormisspellingsomething,thecompilationwillproceedsilently,andmakeanexecutablefilecalleda.out.Ifyouruna.outbytypingthecommand
a.out
itwillprint
Onothersystems,theruleswillbedifferent;
checkwithalocalexpert.
Now,forsomeexplanationsabouttheprogramitself.ACprogram,whateveritssize,consistsoffunctionsandvariables.Afunctioncontainsstatementsthatspecifythecomputingoperationstobedone,andvariablesstorevaluesusedduringthecomputation.CfunctionsarelikethesubroutinesandfunctionsinFortranortheproceduresandfunctionsofPascal.Ourexampleisafunctionnamedmain.Normallyyouareatlibertytogivefunctionswhatevernamesyoulike,but'
main'
isspecial-yourprogrambeginsexecutingatthebeginningofmain.Thismeansthateveryprogrammusthaveamainsomewhere.
mainwillusuallycallotherfunctionstohelpperformitsjob,somethatyouwrote,andothersfromlibrariesthatareprovidedforyou.Thefirstlineoftheprogram,
tellsthecompilertoincludeinformationaboutthestandardinput/outputlibrary;
thelineappearsatthebeginningofmanyCsourcefiles.ThestandardlibraryisdescribedinChapter7andAppendixB.
Onemethodofcommunicatingdatabetweenfunctionsisforthecallingfunctiontoprovidealistofvalues,calledarguments,tothefunctionitcalls.Theparenthesesafterthefunctionnamesurroundtheargumentlist.Inthisexample,mainisdefinedtobeafunctionthatexpectsnoarguments,whichisindicatedbytheemptylist().
Thestatementsofafunctionareenclosedinbraces{}.Thefunctionmaincontainsonlyonestatement,
Afunctioniscalledbynamingit,followedbyaparenthesizedlistofarguments,sothiscallsthefunctionprintfwiththeargument"
.printfisalibraryfunctionthatprintsoutput,inthiscasethestringofcharactersbetweenthequotes.
Asequenceofcharactersindoublequotes,like"
iscalledacharacterstringorstringconstant.Forthemomentouronlyuseofcharacterstringswillbeasargumentsforprintfandotherfunctions.
Thesequence\ninthestringisCnotationforthenewlinecharacter,whichwhenprinted
advancestheoutputtotheleftmarginonthenextline.Ifyouleaveoutthe\n(aworthwhileexperiment),youwillfindthatthereisnolineadvanceaftertheoutputisprinted.Youmustuse\ntoincludeanewlinecharacterintheprintfargument;
ifyoutrysomethinglike
hello,world"
theCcompilerwillproduceanerrormessage.
printfneversuppliesanewlinecharacterautomatically,soseveralcallsmaybeusedtobuildupanoutputlineinstages.Ourfirstprogramcouldjustaswellhavebeenwritten
hello,"
world"
\n"
toproduceidenticaloutput.
Noticethat\nrepresentsonlyasinglecharacter.Anescapesequencelike\nprovidesageneralandextensiblemechanismforrepresentinghard-to-typeorinvisiblecharacters.
AmongtheothersthatCprovidesare\tfortab,\bforbackspace,\"
forthedoublequoteand\\forthebackslashitself.ThereisacompletelistinSection2.3.
Exercise1-1.Runthe'
programonyoursystem.Experimentwithleavingoutpartsoftheprogram,toseewhaterrormessagesyouget.
Exercise1-2.Experimenttofindoutwhathappenswhenprints'
sargumentstringcontains\c,wherecissomecharacternotlistedabove.
1.2VARIABLESANDARITHMETICEXPRESSIONS
ThenextprogramusestheformulaoC=(5/9)(oF-32)toprintthefollowingtableofFahrenheit
temperaturesandtheircentigradeorCelsiusequivalents:
1-17
20-6
404
6015
8026
10037
12048
14060
16071
18082
20093
220104
240115
260126
280137
300148
Theprogramitselfstillconsistsofthedefinitionofasinglefunctionnamedmain.Itislongerthantheonethatprinted'
butnotcomplicated.Itintroducesseveralnewideas,includingcomments,declarations,variables,arithmeticexpressions,loops,andformattedoutput.
/*printFahrenheit-Celsiustableforfahr=0,20,...,300*/
intfahr,celsius;
intlower,upper,step;
lower=0;
/*lowerlimitoftemperaturescale*/
upper=300;
/*upperlimit*/
step=20;
/*stepsize*/
fahr=lower;
while(fahr<
=upper){
celsius=5*(fahr-32)/9;
%d\t%d\n"
fahr,celsius);
fahr=fahr+step;
Thetwolines
/*printFahrenheit-Celsiustable
forfahr=0,20,...,300*/
areacomment,whichinthiscaseexplainsbrieflywhattheprogramdoes.Anycharactersbetween/*and*/areignoredbythecompiler;
theymaybeusedfreelytomakeaprogrameasiertounderstand.Commentsmayappearanywherewhereablank,tabornewlinecan.InC,allvariablesmustbedeclaredbeforetheyareused,usuallyatthebeginningofthefunctionbeforeanyexecutablestatements.Adeclarationannouncesthepropertiesofvariables;
itconsistsofanameandalistofvariables,suchas
Thetypeintmeansthatthevariableslistedareintegers;
bycontrastwithfloat,whichmeansfloatingpoint,i.e.,numbersthatmayhaveafractionalpart.Therangeofbothintandfloatdependsonthemachineyouareusing;
16-bitsints,whichliebetween-32768and+32767,arecommon,asare32-bitints.Afloatnumberistypicallya32-bitquantity,withatleastsixsignificantdigitsandmagnitudegenerallybetweenabout10-38and1038.Cprovidesseveralotherdatatypesbesidesintandfloat,including:
charcharacter-asinglebyte
shortshortinteger
longlonginteger
doubledouble-precisionfloatingpoint
Thesizeoftheseobjectsisalsomachine-dependent.Therearealsoarrays,structuresandunionsofthesebasictypes,pointerstothem,andfunctionsthatreturnthem,allofwhichwewillmeetinduecourse.
Computationinthetemperatureconversionprogrambeginswiththeassignmentstatements
whichsetthevariablestotheirinitialvalues.Individualstatementsareterminatedbysemicolons.
Eachlineofthetableiscomputedthesameway,soweusealoopthatrepeatsonceperoutputline;
thisisthepurposeofthewhileloop
...
Thewhileloopoperatesasfollows:
Theconditioninparenthesesistested.Ifitistrue(fahrislessthanorequaltoupper),thebodyoftheloop(thethreestatementsenclosedinbraces)isexecuted.Thentheconditionisre-tested,andiftrue,thebodyisexecutedagain.Whenthetestbecomesfalse(fahrexceedsupper)theloopends,andexecutioncontinuesatthestatementthatfollowstheloop.Therearenofurtherstatementsinthisprogram,soitterminates.
Thebodyofawhilecanbeoneormorestatementsenclosedinbraces,asinthetemperatureconverter,orasinglestatementwithoutbraces,asin
while(i<
j)
i=2*i;
Ineithercase,wewillalwaysindentthestatementscontrolledbythewhilebyonetabstop(whichwehaveshownasfourspaces)soyoucanseeataglancewhichstatementsareinsidetheloop.Theindentationemphasizesthelogicalstructureoftheprogram.AlthoughCompilersdonotcareabouthowaprogramlooks,properindentationandspacingarecriticalinmakingprogramseasyf